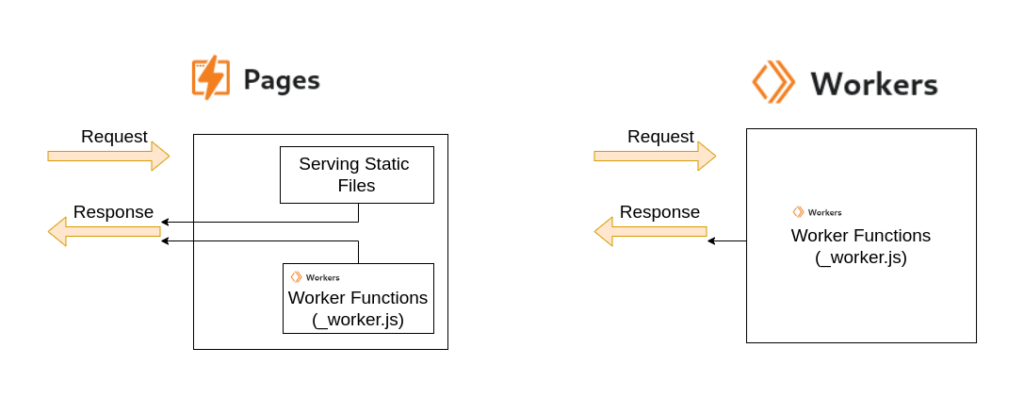
The Challenge:Wildcard Domains on Cloudflare Pages
If you’re building a multi-tenant app, chances are you’ll need to handle wildcard domains (*.example.com). In my case:
• Each subdomain represented a client (e.g., client1.example.com, client2.example.com).
• The frontend was deployed on Cloudflare Pages, using a shared codebase for all tenants.
• The backend dynamically updated branding based on the hostname.
Unfortunately, Cloudflare Pages does not support wildcard domains. This made routing requests for all subdomains a major roadblock.
Adding to the complexity, I needed certain subdomains (like api.example.com and www.example.com) to remain unaffected by any routing changes.
The Solution: Cloudflare Workers to the Rescue
To solve this, I used Cloudflare Workers to rewrite incoming requests dynamically. With just a few lines of code, I:
• Routed wildcard subdomains to my Cloudflare Pages app.
• Excluded specific subdomains from the wildcard routing.
Here’s the final Worker script I used:
Cloudflare Worker Script
export default { async fetch(request) { const url = new URL(request.url); // Subdomains to exclude from wildcard routing const excludedSubdomains = ["api", "email", "portal", "www"]; // Check if the request hostname matches an excluded subdomain if (excludedSubdomains.some(subdomain => url.hostname === `${subdomain}.example.com`)) { // Allow excluded subdomains to pass through unchanged return fetch(request); } // For all other subdomains, rewrite the hostname to point to the Pages app const targetDomain = `your-cloudflare-pages-app.pages.dev`; // Replace with your Pages app domain url.hostname = targetDomain; // Serve the rewritten request return fetch(url.toString(), request); }, };
How It Works
1. Define Exclusions
Subdomains like api.example.com or www.example.com are listed in the excludedSubdomains array. These subdomains bypass the wildcard routing logic.
const excludedSubdomains = ["api", "email", "portal", "www"];
2. Check and Pass Through
If the hostname matches any excluded subdomain, the request is fetched and served as-is.
if (excludedSubdomains.some(subdomain => url.hostname === `${subdomain}.example.com`)) { return fetch(request); }
3. Rewrite for Wildcard Subdomains
Any subdomain not in the exclusion list is rewritten to the Cloudflare Pages app URL.
const targetDomain = `your-cloudflare-pages-app.pages.dev`; url.hostname = targetDomain;
4. Fetch the Updated Request
The Worker fetches and serves the rewritten request.
return fetch(url.toString(), request);
How to Deploy the Worker
Follow these simple steps to set up your Worker:
1. Create the Worker
• Go to Workers & Functions in your Cloudflare dashboard.
• Create a new Worker and paste the code above into the editor.
2. Configure a Wildcard Route
• Under the Routes section, add a route like:
*.example.com/*
3. Test Your Setup
• Visit an excluded subdomain like api.example.com to ensure it’s unaffected.
• Test wildcard subdomains (e.g., client1.example.com) to confirm they’re routed correctly to your Cloudflare Pages app.
Why This Solution is Perfect for Multi-Tenant Apps
This approach is a game-changer if you:
• Run a multi-tenant app where each subdomain represents a client.
• Need to dynamically serve the same frontend with backend logic handling customizations.
• Want specific subdomains (like APIs or admin portals) to bypass routing changes.
Key Benefits of Using Cloudflare Workers
• Wildcard Domain Routing: Easily handle *.example.com requests without native wildcard support in Cloudflare Pages.
• Flexibility: Exclude critical subdomains with ease by updating the exclusion list.
• Scalability: Workers seamlessly scale with your application’s traffic.
Final Thoughts
If you’re deploying multi-tenant apps and struggling with wildcard domains, Cloudflare Workers offer a simple and scalable solution. With just a small script, you can dynamically route requests and keep your app running smoothly.
Have you faced a similar challenge? How did you solve it? Let me know in the comments below!